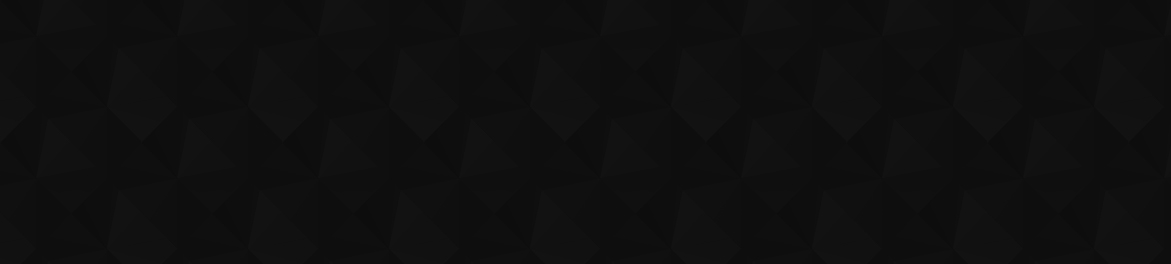
- 141
- 880 769
Josef1
Приєднався 8 тра 2011
How I control QLC+ with a Stream Deck using websockets and Python
Controlling QLC+ with a Stream Deck using websockets and Python involves creating an interactive lighting control setup that bridges sophisticated software control with a user-friendly hardware interface. This setup allows for real-time manipulation of lighting scenes and effects directly from a Stream Deck, a customizable control surface commonly used for live streaming and video production. Here's a concise overview of the process:
QLC+ Configuration: Begin by setting up your lighting scenes, fixtures, and cues within QLC+, a versatile lighting control software. Ensure that QLC+ is configured to accept websocket connections, which will allow external commands to trigger actions within the software.
Websockets Server in Python: Develop a Python script that functions as a bridge between the Stream Deck and QLC+. This script uses websockets to communicate with QLC+, sending commands based on button presses detected from the Stream Deck. Python's websockets library can be used to establish this connection, listening for commands from the Stream Deck and forwarding them to QLC+.
Stream Deck Configuration: Configure your Stream Deck with buttons representing various lighting scenes or commands you wish to execute in QLC+. Each button can be assigned a specific command that correlates with an action or scene in QLC+.
Python Script for Stream Deck Integration: Utilize Python to detect button presses on the Stream Deck. This can be achieved through libraries that interface with the Stream Deck SDK, allowing your script to know when a button is pressed and which command to send over websockets.
Command Mapping and Execution: Map each Stream Deck button to a specific command within your Python script. When a button is pressed, the script translates this into a websocket message, which is sent to QLC+, triggering the corresponding action or scene change.
Run and Test: With everything set up, run your Python script, and test the integration by pressing buttons on your Stream Deck. Each button press should result in an immediate response from QLC+, changing lighting scenes or triggering effects as configured.
This method provides a tactile, quick-response interface for controlling lighting setups, making it ideal for live performances, events, or any application where direct and immediate control over lighting is desired. By leveraging websockets for communication, this setup ensures that commands are relayed in real-time, allowing for seamless interaction between the Stream Deck and QLC+.
Heres the code
import asyncio
from StreamDeck.DeviceManager import DeviceManager
from websocket import create_connection, WebSocketException
# Target WebSocket server URL (to send a message upon button press)
TARGET_WS_URL = "ws://127.0.0.1:9999/qlcplusWS" # Change this to your Qlc+ URL
ws = create_connection(TARGET_WS_URL)
# Function to send a message over WebSocket with error handling
def send_message(TARGET_WS_URL,key):
try:
#ws = create_connection(TARGET_WS_URL)
ws.send(f"{key}|255")
print(f"{key}|255")
except WebSocketException as e:
print(f"WebSocket Handshake: {e}")
except Exception as e:
print(f"Unexpected error: {e}")
# Callback function for button presses with improved error handling
def key_change_callback(deck, key, state):
try:
if state: # Button is pressed
print(f"Button {key} pressed")
# Now also send a message to the target WebSocket server
send_message(TARGET_WS_URL,key)#need to format the messahge to give the button number here
else: # Button is released
print(f"Button {key} released")
except Exception as e:
print(f"Error handling button {key} press/release: {e}")
# Main function remains the same
def main():
stream_decks = DeviceManager().enumerate()
if not stream_decks:
print("No Stream Deck found.")
return
deck = stream_decks[0]
print("Found Stream Deck")
deck.open()
deck.reset()
deck.set_key_callback(key_change_callback)
print("Press buttons on your Stream Deck. Press Ctrl+C to quit.")
try:
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
loop.run_forever()
except KeyboardInterrupt:
print("Exiting...")
finally:
deck.close()
ws.close()
if __name__ == "__main__":
main()
QLC+ Configuration: Begin by setting up your lighting scenes, fixtures, and cues within QLC+, a versatile lighting control software. Ensure that QLC+ is configured to accept websocket connections, which will allow external commands to trigger actions within the software.
Websockets Server in Python: Develop a Python script that functions as a bridge between the Stream Deck and QLC+. This script uses websockets to communicate with QLC+, sending commands based on button presses detected from the Stream Deck. Python's websockets library can be used to establish this connection, listening for commands from the Stream Deck and forwarding them to QLC+.
Stream Deck Configuration: Configure your Stream Deck with buttons representing various lighting scenes or commands you wish to execute in QLC+. Each button can be assigned a specific command that correlates with an action or scene in QLC+.
Python Script for Stream Deck Integration: Utilize Python to detect button presses on the Stream Deck. This can be achieved through libraries that interface with the Stream Deck SDK, allowing your script to know when a button is pressed and which command to send over websockets.
Command Mapping and Execution: Map each Stream Deck button to a specific command within your Python script. When a button is pressed, the script translates this into a websocket message, which is sent to QLC+, triggering the corresponding action or scene change.
Run and Test: With everything set up, run your Python script, and test the integration by pressing buttons on your Stream Deck. Each button press should result in an immediate response from QLC+, changing lighting scenes or triggering effects as configured.
This method provides a tactile, quick-response interface for controlling lighting setups, making it ideal for live performances, events, or any application where direct and immediate control over lighting is desired. By leveraging websockets for communication, this setup ensures that commands are relayed in real-time, allowing for seamless interaction between the Stream Deck and QLC+.
Heres the code
import asyncio
from StreamDeck.DeviceManager import DeviceManager
from websocket import create_connection, WebSocketException
# Target WebSocket server URL (to send a message upon button press)
TARGET_WS_URL = "ws://127.0.0.1:9999/qlcplusWS" # Change this to your Qlc+ URL
ws = create_connection(TARGET_WS_URL)
# Function to send a message over WebSocket with error handling
def send_message(TARGET_WS_URL,key):
try:
#ws = create_connection(TARGET_WS_URL)
ws.send(f"{key}|255")
print(f"{key}|255")
except WebSocketException as e:
print(f"WebSocket Handshake: {e}")
except Exception as e:
print(f"Unexpected error: {e}")
# Callback function for button presses with improved error handling
def key_change_callback(deck, key, state):
try:
if state: # Button is pressed
print(f"Button {key} pressed")
# Now also send a message to the target WebSocket server
send_message(TARGET_WS_URL,key)#need to format the messahge to give the button number here
else: # Button is released
print(f"Button {key} released")
except Exception as e:
print(f"Error handling button {key} press/release: {e}")
# Main function remains the same
def main():
stream_decks = DeviceManager().enumerate()
if not stream_decks:
print("No Stream Deck found.")
return
deck = stream_decks[0]
print("Found Stream Deck")
deck.open()
deck.reset()
deck.set_key_callback(key_change_callback)
print("Press buttons on your Stream Deck. Press Ctrl+C to quit.")
try:
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
loop.run_forever()
except KeyboardInterrupt:
print("Exiting...")
finally:
deck.close()
ws.close()
if __name__ == "__main__":
main()
Переглядів: 238
Відео
Making an intertwined wedding ring
Переглядів 270Рік тому
Making an intertwined wedding ring to work out approximated sizes of each individual ring
Scanning the top of a Moto Guzzi Key to make a replica
Переглядів 118Рік тому
Using a 3d scanner and CAD to replicate a Motor Guzzi ignition key top.To the best of my knowledge the plastic tops are not available anymore. So I decided to make a file that could be 3d printed thevideo is that process
Moto Guzzi Custom Build- cafe racer
Переглядів 1,6 тис.Рік тому
Starting my bike for the first time after a full rebuild. Its a 1981 Moto Guzzi Lemans II with a few modifications, Its taken 18 months on and off to get to this point. I was excited and a little apprehensive to see if it would start up. I think the 40mm Lafranconi exhaust note is lovely.
Slow motion of jewellery been cooled after soldering
Переглядів 2052 роки тому
Slow motion of jewellery been cooled after soldering
3D Scanning a ring to make a shaped wedding band
Переглядів 2253 роки тому
3D Scanning a ring to make a shaped wedding band . The ring is converted to a stl file which can be inported into Rhino then modeled agaist . I will make a full video of the process when I get a bit of spare time.
how to lost wax cast a wax crowskull
Переглядів 1623 роки тому
Short video on lost wax casting a crows skull
DIY Magnetic pin polishing machine
Переглядів 14 тис.3 роки тому
A quick overview of the DIY Magnetic pin polishing machine that I have been putting together .
Magnetic pin polisher slow motion
Переглядів 3183 роки тому
Magnetic pin polisher slow motion. You can see the way the pins work the metal to clean the surface shot at 960 FPS
Making a Mixed Martial Arts Silver and Gold Blackbelt Award Ring
Переглядів 2344 роки тому
Making bespoke rings and pendants for WBBA . the process of designing the items moulding soldering casting polishing and finishing.
Setting a heart shaped pendant
Переглядів 2254 роки тому
Tribute to those lost through setting accidents.
setting an Eternity ring
Переглядів 2,3 тис.5 років тому
Setting an Eternity ring using 2.4mm stones into the gold ring. I used a 2.3mm ball bur and an engraving tool fitted with a concave bit to round out the settings.
Converting a Revo 540 CX mill to run on Mach 3 (Part 1)
Переглядів 1,4 тис.5 років тому
Converting a Revo 540 CX mill to run on Mach 3 (Part 1)
Running mach and a smooth on revo mill
Переглядів 4316 років тому
Running mach and a smooth on revo mill
Rhino jewellery flow along a surface bead tutorial
Переглядів 2 тис.6 років тому
Rhino jewellery flow along a surface bead tutorial
Hi, I really want to try to do this tutorial, and it's not working because Rhino 8 does not have the tspline splitcurve option, do you know where I can find it? Do I have to use grasshopper?
You could use the split command and set it to point in the command line and do each intersection manually. Tsplines was discontinued. I've not used rhino 8 yet but maybe there is a similar command in the sub d menu ?
thanks and how can I do textureing in rhino - Sculptris is not longer available and zbrush core mini does not allow the import of obj files. I got stuck there. Much appreciated! I really like this tutorial!
Tutorial make jig sharpening please
I have a better jig when I get chance I will film that for you
Are those mechanical switches?
I am Devendra can you tell me in the middle tumbler which brown soil for for polishing you are using
its wallnut shells and rouge
@josef1 for a nice slim bezel edge. What minimums would you work to when modelling in Rhino and printing?
))
Hello, I want to ask where you can download the revo mill program that controls the machine
Its mach3 www.machsupport.com/software/mach3/
@@Josef1cnc Thanks
THANK YOU VERY USEFUL
thank you
Wow! Very nice.
nice work , sounds great
please magnet pursaches online link
first4magnets™ F205N-ZN-2 Magnet Expert® 20mm dia x 5mm thick x 5.2mm c/s Zinc Plated N42 Neodymium Magnet - 8.3kg Pull ( North ) ( Pack of 2 ) amzn.eu/d/iKRrbbO. Something like this you have to be able to swap the poles over
Hey Joe, I just started the mod, I'm still buzzing the wires with a multimeter and writing down all the pins. It's going very well so far. I was wondering if you also noticed that A-axis and Z-axis share the same dir+ and step+ signal, it seems like only dir - of both Axis have discreet connections. I was wondering if you separated their signals or you if you left them as is. I'm worried about magic smoke escaping if I power them to the new controller. Any advice? Also did you keep the original power supply, I wanted to keep it because it besides being wired already they have the status LEDs shining through the Metal column holes, blinky leds make me happy I want to keep it 🤣. If you did keep it, what pins turn on the power Supply? Thanks Joe
That's great , I can't remember from memory and don't want to say he wrong thing but they may be switched by negative which would mean all the positives goto the same place. I will have a look tomorrow , I think it's like that for all axis's . I swapped the power supply out to make things easier for me also because we have 240v here . Again I will have a check tomorrow and let you know what voltage I used .
Great thanks, I will update you, I will try to keep the PSU it just fits well in there. I have to figure out how it's turned on. If I can't I will just replace it with something smaller. It's amazing how this company managed to hide the schematics for something for so long my mill has a stamp of 2007 manufacturing date, yet you google the parts nothing comes up other than surestep Drives and the Steppers everything else is a blur.
Your sounds are note clr.... plzz make clr for the next time..... clsses are good.. 😊
Yeah I know I need to get a new mic !
How can I get in contact with you?
If you goto ua-cam.com/users/Josef1cncabout you should find an email address
Hi! I am helping a jeweler friend troubleshoot his 540C. Any good guides/literature you may have? Seems there is no longer any support for this machine and as of now, its just a giant paperweight. Thanks!
The problem is the software to run it is now obsolete from Gemvision Matrix. I converted it to run on mach3/4 by fitting an ethernet smoothstepper and rewiring some of it. It works great, I use deskproto to do the toolpathing. A version of this software was originally used and you can unzip the files from it and get the G code if you have existing models. You could use any pathing program just needs set up to work with Mach and set up a postprocessor .What is the problem with the mill ?
@@Josef1cnc Thanks for the reply. The machine has been working fine and in the middle of a job, it stopped. Symptoms now are when the mill is fired up, it will not home any of the axes. “Unable to find home” with no axes moving at all. I am able to use the software to move the x-axis (both directions) and the a-axis (clockwise and counter-clockwise). The y-axis will only move forward, even when commanded to move aft and the erroneously thinks it’s hitting the limit switch. The z-axis will not move at all; sits there silently (no grinding or buzzing noises) but the software shows movement. The mill has not been taken apart since it stopped working. I checked all the fuses and they are all in good order.
@@Josef1cnc thanks for the video, we're you able to use the existing drivers? I was thinking the same as you, I have the smoothstepper ethernet version as well as a pmdx 126 breakout box. I was wondering if you swapped the drivers to geckodrives or something else. What Break out box did you use with your smoothstepper? Thanks you gave courage to start this mod. Thanks again Cheers from Canada.
@@Pixelvore1 I used the drivers in the Revo they were fine I google the model to get wiring diagrams and it was simple enough. I used a DB25 D-SUB Male 25Pin Plug Breakout Board with screw terminals off ebay and used FEMALE LPH26-to-DB25 FEMALE cables from the smooth stepper to the breakout boards, I did have a smoothstepper breakout board but it was a bit big for where i wanted to fit it. I also used a couple of arduino type relays to switch the spindles on also i rewired the home switches to the smooth stepper which worked great.If you get stuck give me a shout I will try and help Cheers Joe
@@Josef1cnc Man, good to know. You Saved me a bunch of money, I have been looking into geckodrives because I thought those drives in the revo were proprietary to gemvision board. I will dive into this in a couple of weeks. It's very good to know you got it working. Congratulations and thanks for offering me the help, I will definitely get stuck somewhere. I'm glad to know at least one person on earth converted the Revo to run on Mach3/4. Cheers Joe.
Thanx
Thanx
Thanx
boltgen ?
www.food4rhino.com/en/app/boltgen-manuals-and-help-rhinoceros-windows-and-mac
Thanx
Thank you sir , do we need flashback arrestor for oxygen generator ?
I don't on mine I don't think, I will check when I'm in work, I do have one on the propane tank I think
Did you have to round it out because the stone was a little bigger than the opening?
Yes the stones tend to be different sizes so you take away metal until they fit in
hello awesome work, i noticed it didnt look like you cut any seats fot the stones, just opened the hole up with a ball burr and pushed the prongs down?
adammm
At the moment I'm also working on a magnetic tumbler for jewellery and maybe small art pieces size slave bracelet squared but having a hard time choosing an engine. What RPM and Wattage would you recommend?
The details are in the video on the motor I think it's 1/3 of a horsepower or maybe 1/2 I will check. I can't remember the rpm varies on what you have in it I've found about 800 is a good starting point on mine
@@Josef1cnc I did find the section where you showed the motor's technical details but you did mention that it was quite a bit overpowered ;) At the moment I'm pondering between 2 motor types. 1 with a max rmp of 3000 (effectively 2750) and 2000 rmp (effectively 1750) I know I can use a frequency dimmer to control the speed but I will also lose torqe. If 1750 would be way more than enough than that motor will be my choise, otherwise I should turn towards the 3000 but there are few people giving experience with softer metals like silver. Hence the question. What is the max rmp you use?
@@rolfvanderbijl The motor runs to 2760 on the tag I use between 30% and 60% of that anything higher doesn't seem to work for me so that just over 1500 rpm I think. I have recently tried 0.2mm pins in it and have to run slower around 40% for it to work nicely. I guess the magnet strength, size of rotor disc and size of container all play into the equation but I get pretty ok results. I think been able to change the speed seems to be the key so you can dial it in you can see the pins dancing when you get it right I have another vid of this ua-cam.com/video/SvQnJ7IGCvA/v-deo.html&ab_channel=Josef1. I was thinking of gluing a funnel into the middle of the bowl to push everything into the stream of pins but not got round to that yet.
@@Josef1cnc Seen the slip speed of motors is lower than the theoretical speed I then think I should go towards 3000, also seen I might use the polisher for different purposes like small steel items like knife blanks and alike. A propper fequence regulator should then solve the issue of dialing down speed I guess. You might want to have a look at 3D printing for your proof of concept regarding the funnel concept. Take a known sized bucket and print an insert to try it out with. Besides a funneltype shape (which has an empty space in the centre), it might be worth looking into circular V groove which follows the path of the rotating magnets and optionally a circular wave shape to push the pins up and down along their path forcing them act more agitated. But ofcourse you can experiment with different shapes AND textures to get the pins moving around more. I couldn't find an example image quickly but if you have questions or arn't that familiar with 3D-printing please let me know and we might be able to help eachother out further.
@@rolfvanderbijl That's a good idea I will have a go at cadding something
merci
You should do ASMR mate. Good tutorial btw.
HELLOOO!
Hello 😊
Good
It seems rhino sucks at filleting... fusion 360 seems to do a nice job on the other hand
Thank you very much. You made my day
very good bravo
Hey Josef, the video is great. Have you tried Casting such a fine screw thread? Or does that need to be manually threaded? Thank you for sharing your knowledge.
I have printed and cast threads but not that small I would just use a die to cut them maybe cast the screw blanks
@@Josef1cnc thank you for replying. I want to design a bangle with a hinge on one side & some type of hidden clasp on the other side. I would like to cast as much as possible than do manual work. Do you think you can guide me through it? Sometimes I'm unsure what can be Casted and what can't.
@@Narz1981 the only bit you can't cast possibly is the hinge pin and the tongue on the clasp that secures the bangle
ua-cam.com/video/Ve_mWxy8o10/v-deo.htmlsi=3MfOnE93GWlbFhVk
Nice one, Joe!
i have a revo 540 a and i would like to run as mach 3. how much could it cost to do it. Thanks
What is the necessity to convert this machine from its original system to mach3? I'm not a Revo user, just curious.
Thanks for the tutorial, but at this step 1:42 my command history window says: Split failed, objects may not intersect or intersections may not split object. :(
If you explode the model not the pipe then try the spit this sometimes shows up the problem area.
Okey I fixed it. Just by exploding both the object and the splitting object (apparently there was some problem with their geometry that made the split not possible without exploding them first)
you dont show thw tool bar... sorry
Is this Clayoo?
Nope just rhino it's sub select
@@Josef1cnc do you work in RhinoGold?
@@emildiaco78 no I don't
what cnc mill do you use?
That one was a minitech 3.
5 axis?
@@emildiaco78 no 4 axis I cut in 2 halfs to get the hollow then glued together and did the outer rotary finish if I remember
Thank you. Do you use 5 axis cnc in present?
@@emildiaco78 no but I did build one toolpathing it was a pain so didn't go any further with it then printers come along
render
Wow. This UV from object just saved my ass.
hi, where to download the ProtoWizard software. please advice.
You can't the software has been discontinued maybe look at deskproto
If you have it. Please share it with me. Thanks
Please share it with me sir
I like
Well done. Some of these rhino commands are out of this world
Nice job, mate!
Thanks mate!
when I import my rhino file into sculptris, the ring has weird lines forming inside it
Ssss....sssss...ssss....
how come you have to split the twig curves to do a flow along surface? and is there a nontsplines command for that now? or do you have to tediously go one by one?
hey awesome one, I hope you are doing well! what happened to the site?!
This is very cool! Is the probe supposed to deflect that much?
No it was on a rubber tube while I was setting things up so I didn't crash anything!